Quickstart: React
Cypress Component Testing is currently in beta.
To follow along with this guide, you'll need a React application.
The quickest way to get started writing component tests for React is to use one of the frameworks out there that can create a React project for you, like Create React App, or Vite. In this guide, we will use Vite as it's quick to get up and running.
To create a React project with Vite:
- Run the scaffold command
npm create vite@latest my-awesome-app -- --template react
- Go into the directory and run
npm install
cd my-awesome-app
npm install
- Add Cypress
npm install cypress -D
- Open Cypress and follow the Launchpad's prompts!
npx cypress open
Configuring Component Testing
Whenever you run Cypress for the first time in a project, the app will prompt you to set up either E2E Testing or Component Testing. Choose Component Testing and step through the configuration wizard.
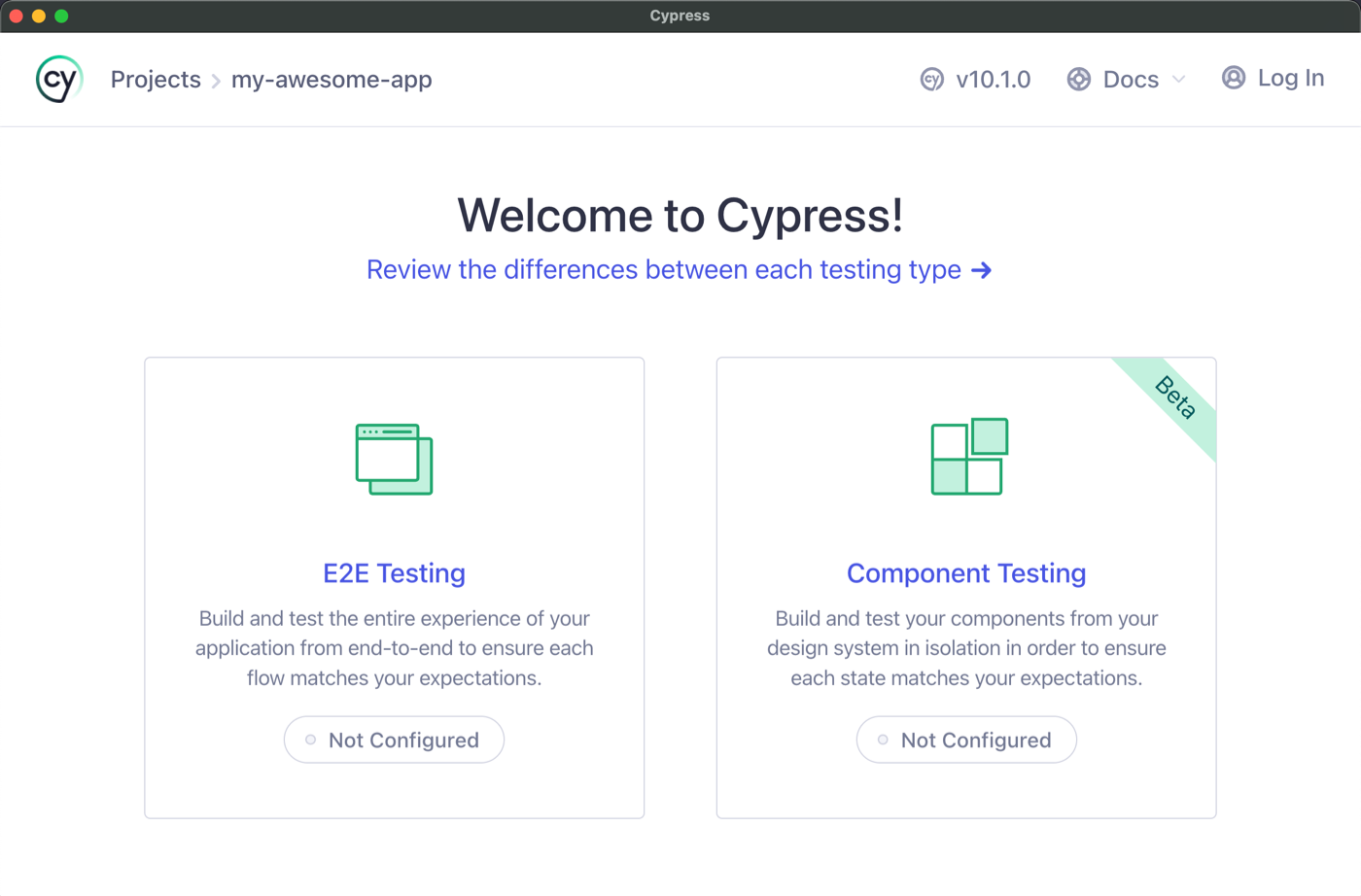
Choose Component Testing
The Project Setup screen automatically detects your framework and bundler, which is React and Vite in our case. Cypress Component Testing uses your existing development server config to render components, helping ensure your components act and display in testing the same as they do in production.
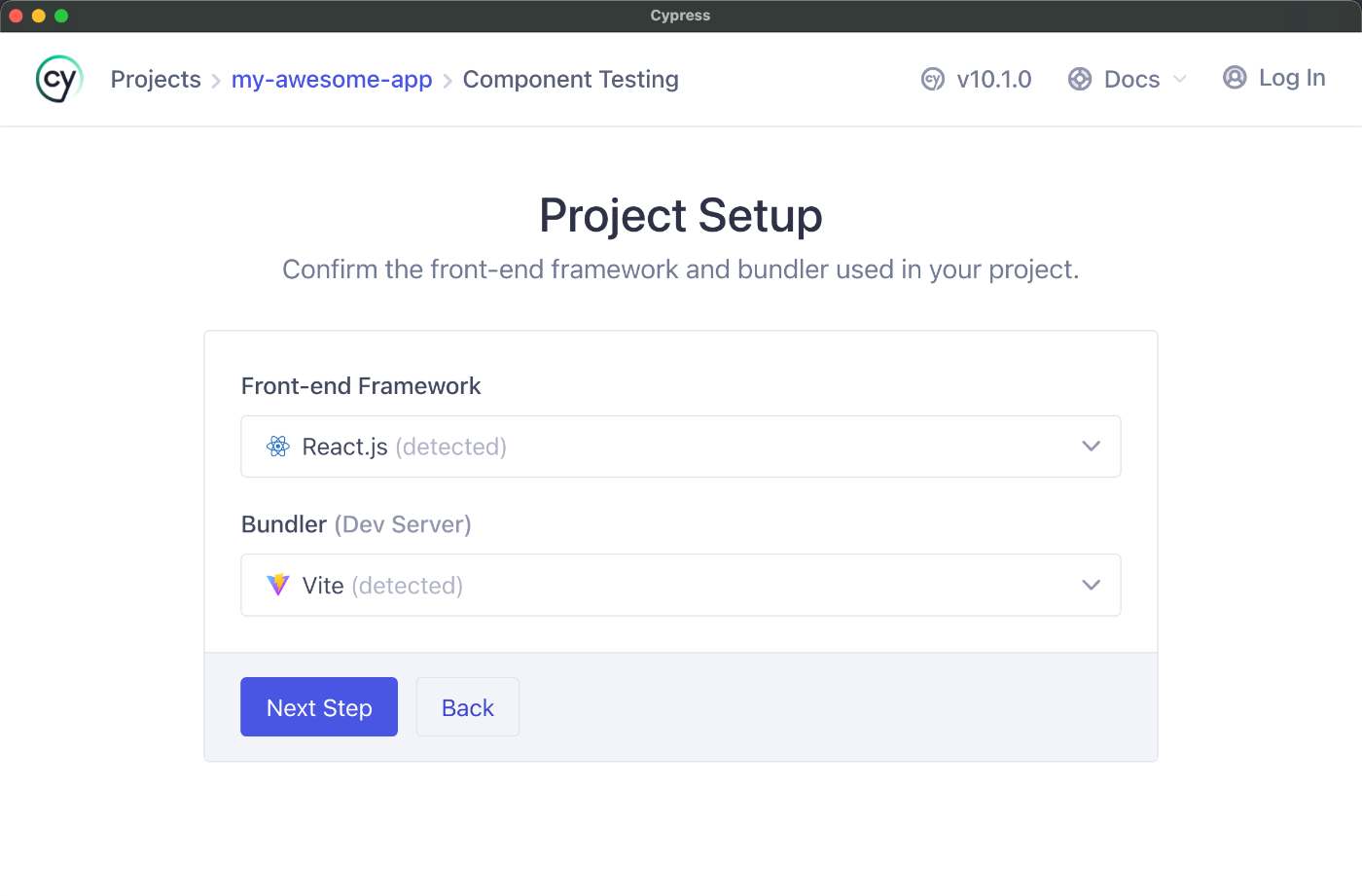
Next, the Cypress setup will detect your framework and generate all the necessary configuration files, and ensure all required dependencies are installed.
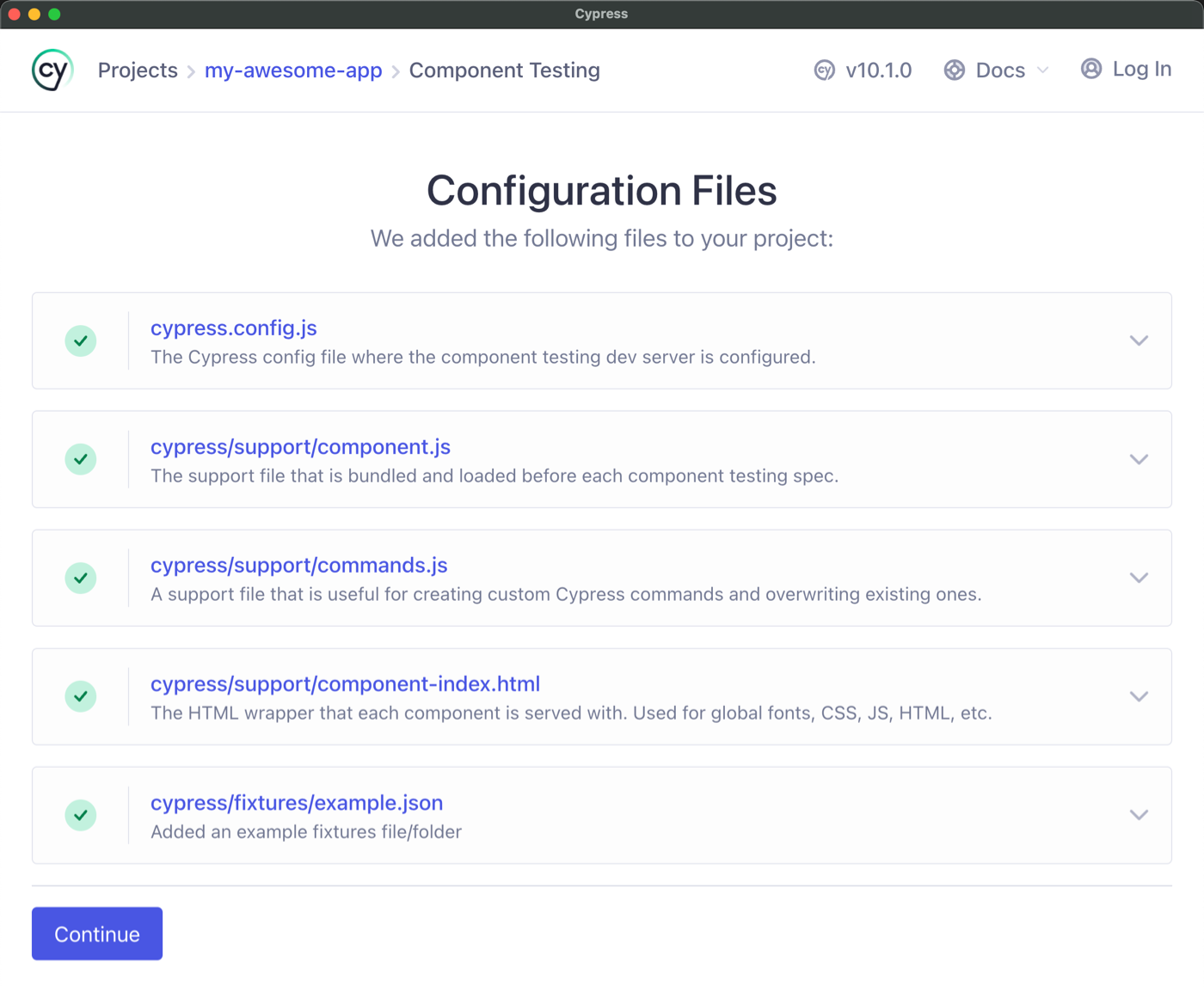
The Cypress launchpad will scaffold all of these files for you
After setting up component testing, you will be at the Browser Selection screen.
Pick the browser of your choice and click the "Start Component Testing" button to open the Cypress app.
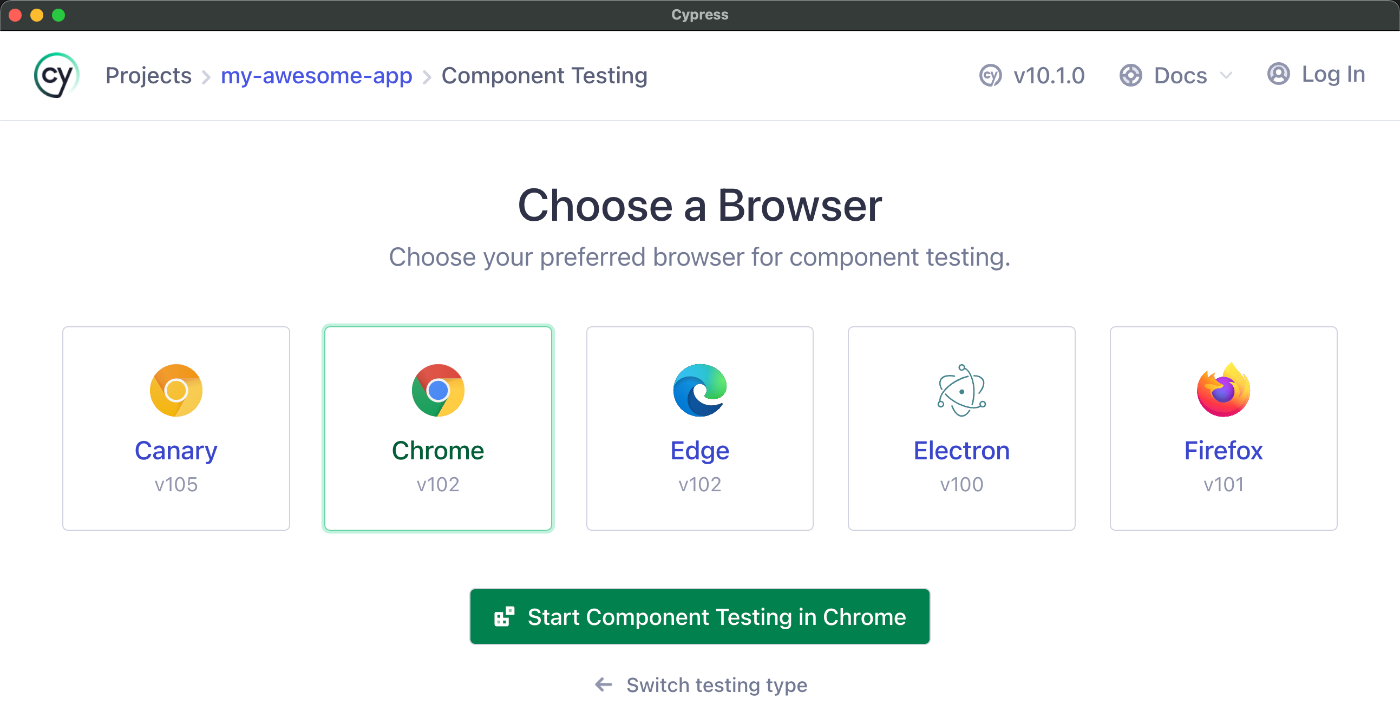
Choose your browser
Creating a Component
At this point, your project is set up but has no components to test yet.
In this guide, we'll use a <Stepper/>
component with zero dependencies and one
bit of internal state -- a "counter" that can be incremented and decremented by
two buttons.
If your component uses providers, network requests, or other environmental setups, you will need additional work to get your component mounting. This is covered in a later section.
Add the Stepper component to your project:
import { useState } from 'react'
export default function Stepper({ initial = 0 }) {
const [count, setCount] = useState(initial)
return (
<div>
<button aria-label="decrement" onClick={() => setCount(count - 1)}>
-
</button>
<span data-cy="counter">{count}</span>
<button aria-label="increment" onClick={() => setCount(count + 1)}>
+
</button>
</div>
)
}
Next Steps
Next, we will learn to mount the <Stepper />
component with the mount command!